Object-Oriented Programming (OOP) is foundational in software engineering, making code modular, scalable, and maintainable. Testing is also of prime importance in software development in that it guarantees reliability and correctness for applications. The features offered by OOP support several testing activities, including Unit Testing, Integration Testing, and Test-Driven Development (TDD). This article discusses how OOP eases the path for these different testing strategies and why it is one of the preferred ways to enable software development for robustness.
The unit testing is a practice of testing each component or method of a given application in isolation. OOP promotes unit testing with the addition of the following concepts:
- Encapsulation:This naturally places all the data and behavior for OOP inside the class. From this, the developer can work on exactly one specific method of a certain class without any external dependencies within that method. Thus, one can keep an individual working in a proper condition and therefore only affect the individual actions.
- Modularity: OOP promotes breaking applications into reusable classes and methods, allowing independent testing of specific functionalities.
- Mocking and Dependency Injection: Often while unit testing, a test requires isolation of components from dependencies. OOP enables dependency injection, using mock objects and stubs to test classes without external module reliance.
- Polymorphism and Interfaces: Polymorphism and interfaces let programmers use test doubles for unit tests without relying on specific service classes.
Developers use OOP features with popular unit-testing frameworks like JUnit (for Java), PyTest (for Python), and NUnit (for .NET) to test class behaviors effectively.
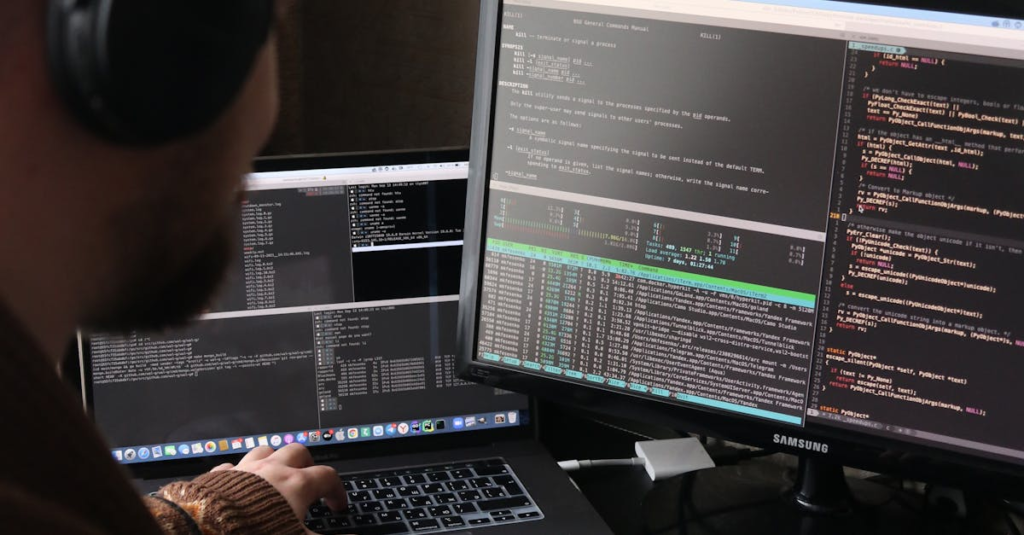
Seamless Integration Testing
Integration testing verifies how multiple components interact within an application. OOP principles aid in integration testing by:
- Clear Module Boundaries: Here, the purpose of classes is to manage data flow, permitting open communication and feedback among modules.
- Encapsulated Behaviors: OOP enforces boundaries, allowing integration testing to focus on interactions between objects with internal state remaining intact.
- Inheritance and Reusability: Base classes tend to decrease redundant code, thereby ensuring Integration tests remain consistent and maintainable.
- Mocking and Faking: OOP provides the capability of performing integration testing through substituting real dependencies with mock implementations for more accurate testing.
For integration testing, widely used tools include Selenium for UI testing, Postman for API testing and TestNG for Java applications; all of these are very much in line with OOP methodologies.
Test-Driven Development (TDD) involves writing tests before code implementation, ensuring better software quality and design through OOP. Another development strategy that TDD is complemented by is OOP:
- Incremental Development: All systems are broken down into testable, small modules that correspond with, and thus are well suited to, OOP’s modularity.
- Refactoring with Confidence: Encapsulation and abstraction in OOP ensure that changes to code can be made without altering any behavior pertinent to external interaction, hence allowing reliable test cases.
- Encapsulation for Predictable Behavior: Classes provide well-defined groups, serving as predictable units when they follow the TDD approach for role-based testing.
- Encourages Maintainability: Well-structured OOP code makes a system self-documenting, consequently easing future modifications and maintenance.
JUnit (for Java), RSpec (for Ruby), and xUnit (for C#) support test-driven environments without compromise, ensuring the highest level of code quality and design.
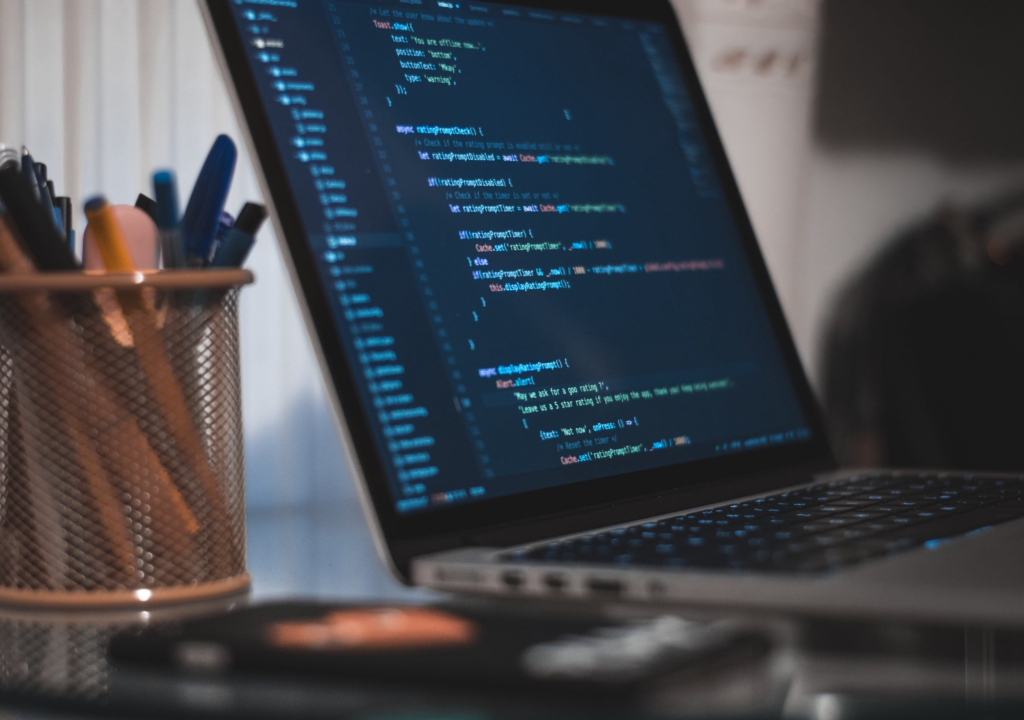
A close-up of a laptop screen displaying code in a development environment, symbolizing programming and software development.
Conclusion
OOP enables structured, modular, and reusable code, optimally supporting Unit Testing, Integration Testing, and Test-Driven Development. These principles of encapsulation, polymorphism, and modularity further empower developers to write testable, manageable, and scalable applications. Using OOP with proper testing ensures software reliability, performance, and adaptability in a constantly changing technology environment.
Kindly visit Jazz Cyber Shield to get more behind-the-scenes information on O.Q., Testing Methodology, and software development best practices.
This article helped me finally connect the dots between OOP and effective testing. The Jazz Cyber Shield blog makes complex topics feel simple.