Inheritance is one of the most powerful and widely applied concepts in Object-Oriented Programming (OOP). It acts as a primary means of Code Reusability in OOP so that applications can be built that are scalable, maintenance-friendly, and performance-efficient. Thus, knowledge of how inheritance helps with code reusability is essential for writing clean and modular code, eliminating redundancy, and encouraging organized software architecture. In this article, we will discuss the significance of inheritance, code reusability based on inheritance, and the best practices for utilizing this feature in programming.
Understanding Inheritance in OOP
Code Reusability in OOP means that one class, called a child class or subclass, can derive properties and behavior from another class, called the parent class or superclass. This allows a child class to inherit the attributes and methods from a superclass, eliminating the necessity of code duplication. These hierarchical relationships not only ease the maintenance of code but also put into place polymorphism and extensibility.
For example, consider a simple hierarchy in Python:
class Vehicle:
def __init__(self, brand, model):
self.brand = brand
self.model = model
def display_info(self):
return f”Brand: {self.brand}, Model: {self.model}”
class Car(Vehicle): # Car inherits from Vehicle
def __init__(self, brand, model, doors):
super().__init__(brand, model)
self.doors = doors
def display_info(self):
return f”Brand: {self.brand}, Model: {self.model}, Doors: {self.doors}”
car = Car(“Toyota”, “Corolla”, 4)
print(car.display_info())
Moreover , the Car class inherits the brand and model attributes and the display_info() method from the Vehicle class. Instead of rewriting the same properties, we reuse the existing structure, making the code more efficient and readable.
How Inheritance Enhances Code Reusability
1. Avoiding Code Duplication
The inheritance takes all redundant features out by defining the properties in the base class because inheritance is only between the classes with the same light of common properties or behaviors. Thus, one change has to effect those enhancements only at the parent place, thus being eminent to all the child classes.
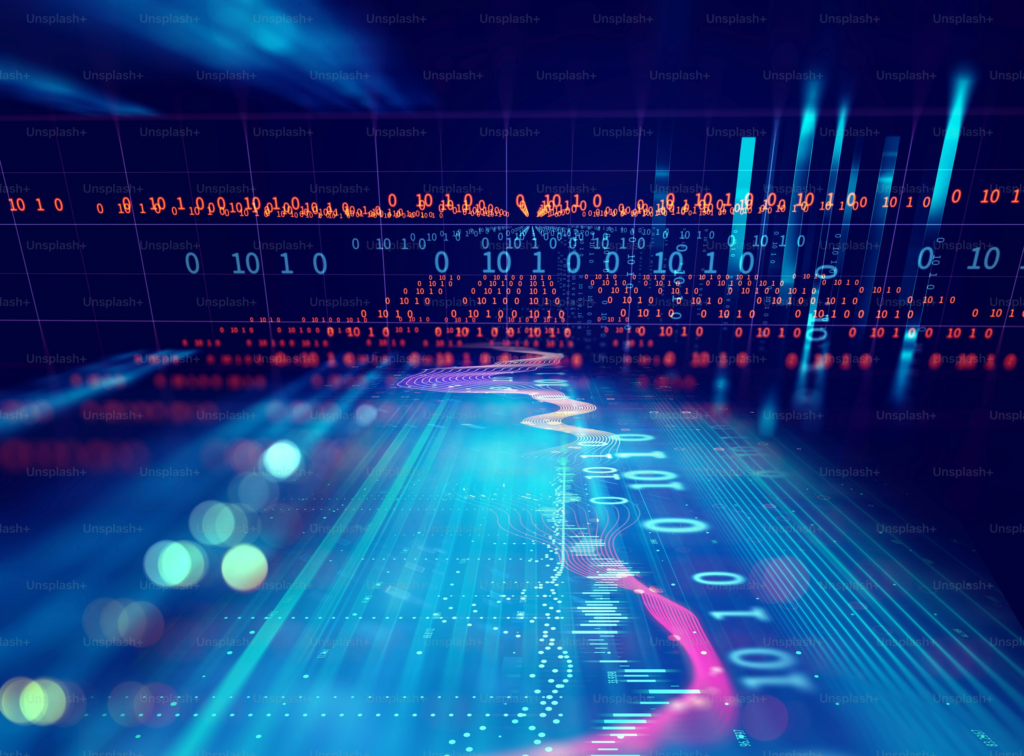
2. Encapsulation and Maintainability
By amalgamating mutual logic in a primitive class, inheritance actually promotes encapsulation. this results in a neat and tidy codebase that would, preferably, be easier to maintain, debug, and extend. Whenever a bug in a shared method is found, fixing the method in the parent class will fix the same bug in all derived classes automatically.
3. Extensibility and Scalability
They can make children classes with extensions in parent classes using inheritance without modifying code that already exists. It makes applications scalable because adding new features will not disturb the already existing structure.
4. Polymorphism for Flexible Implementations
When polymorphism is invoked in combination with inheritance, it allows for child classes to override or even extend the methods of a parent class. this feature welcomed added flexibility across the board. By doing this, various classes can maintain their own behaviors while having a common interface; thereby, it responded well all along.
For instance:
class Animal:
def make_sound(self):
return “Some generic sound”
class Dog(Animal):
def make_sound(self):
return “Bark”
class Cat(Animal):
def make_sound(self):
return “Meow”
def animal_sound(animal):
print(animal.make_sound())
animal_sound(Dog()) # Output: Bark
animal_sound(Cat()) # Output: Meow
Here, different child classes override the make_sound() method, demonstrating polymorphism while still reusing the base class structure.
Best Practices for Using Inheritance Effectively
- Use Inheritance Only When Necessary: The issue of deep hierarchy making maintenance tedious arises from heavy usage of inheritance. In cases where inheritance is not absolutely necessary, consider composition instead.
- Keep Base Classes Generalized: A good base class only includes functionalities that subclasses have in common. It does not contain any function peculiar to any one subclass.
- Leverage Super Calls: Call the superclass methods from within the inheriting class to appropriately exploit inherited functionality.
- Avoid Circular Dependencies: The inheritance structure must logically flow in a hierarchical arrangement to prevent circular dependencies, which are the areas from which debugging is executed.
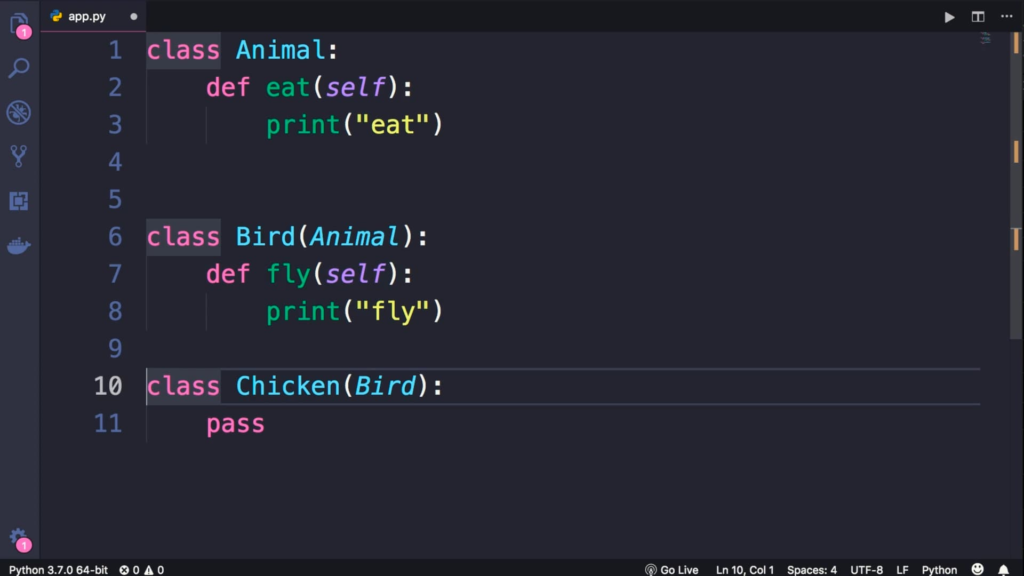
Conclusion
Inheritance stands to be a keystone for the Code Reusability in OOP paradigm. With the help of the base classes, it allows developers to define common functionality and with the help of the derived classes, extend them. Hence, this avoids redundancy, encourages maintainability, and provides a pathway for scalability. When done properly, this creates a more organized and efficient manner in coding, thus facilitating better software development and maintenance.
For some extended discussions regarding programming and technology, visit Jazz Cyber Shield and check out our latest articles on software development and best coding practices.