An OOP is an excellent paradigm of the effectiveness in writing modular, reusable, and maintainable code. Unfortunately, some developers (mostly beginners) may have certain common mistakes that lead one to have code that becomes inefficient, complicated, or even unmaintainable. This article explores these common mistakes, their effects, and best practices to avoid them.
1. Not Following the SOLID Principles
One of the most fundamental mistakes in OOP is neglecting the SOLID principles, which are:
- Single Responsibility Principle (SRP): A class should have only one reason to change.
- Open/Closed Principle (OCP): Software entities should be open for extension but closed for modification.
- Liskov Substitution Principle (LSP): It is just like we want to relax when designing subtypes.
- Interface Segregation Principle (ISP): Because they don’t learn, clients shouldn’t be forced to use interfaces.
- Dependency Inversion Principle (DIP): High-level modules should not depend on low-level modules except on abstractions.
How to Avoid This Mistake
- Stick to the SOLID principles while constructing classes.
- Firstly, consistently audit your design for any breach of those principles.
- Secondly, employ design patterns to lean towards the SOLID principles.
2. Overuse or Misuse of Inheritance
While inheritance represents one of the OOP’s pillars, it is instead used often out of turn, inflicting overly deep subclass chains that surrender much of the benefits of flexibility and maintainability.
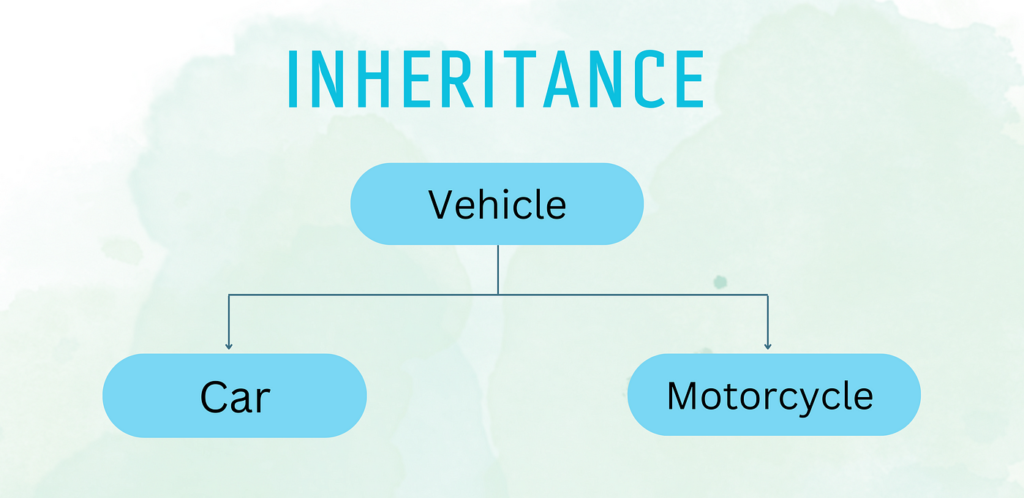
How to Avoid This Mistake
- Composition should be preferred to inheritance; use interfaces and dependency injection for great flexibility instead of a deep inheritance tree.
- Hierrarchies of classes should be kept shallow to improve flexibility and maintainability.
- Use abstract classes and interfaces where appropriate.
3. Tightly Coupled Classes
Classes that are either highly connected or depend heavily on each other’s implementation will be changed only with painstaking effort, since alterations in one of the classes could very well trigger changes in others.
How to Avoid This Mistake
Dependency injection should be used to lessen the direct dependence of classes on each other. Firstly, depend on interfaces, not concrete implementations. Furthermore, use design patterns like the Factory Pattern or Dependency Injection for better decoupling. Ultimately, this approach enhances flexibility and maintainability.
4. Ignoring Encapsulation
Encapsulation hides a class’s internal state and restricts access via well-defined interfaces. However, many developers expose internal state; consequently, maintenance becomes harder, furthermore, system reliability suffers, and ultimately, code quality declines.
How to Avoid This Mistake
- Make instance variables private and provide controlled access to them through getter and setter methods.
- Use the access modifiers – private, protected and public – wisely.
- Do not expose unnecessary internal details of the class.
5. Violating the Law of Demeter
The law of Demeter states that an object should only interact with its immediate dependencies, not with the dependencies of its dependencies.
How to Avoid This Mistake
- Adhere to the “Tell, Don’t Ask” concept of mag natively coordinated object and object task. Do not fetch data from an object and work on it, thereby minimizing any method chains like obj.getA().getB().getC().
- Use appropriate setting instead of allowing the internal dependencies to be open to the world.
6. Improper Use of Static Methods and Variables
Static members can be useful, but overusing them can lead to code that is difficult to test and maintain.
How to Avoid This Mistake
- Do not use static methods for instance-specific operations.
- Be wary of the potential side effects that static variables can create.
- Prefer using dependency injection over static utility classes.
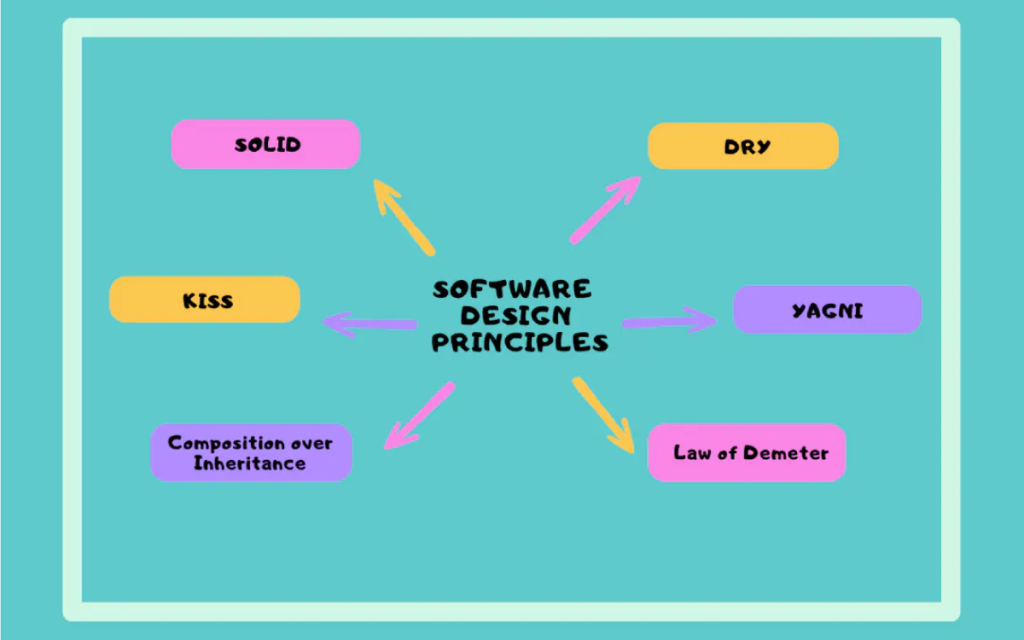
7. Writing Large, Monolithic Classes
Large classes with too many responsibilities violate the Single Responsibility Principle and make code harder to manage.
How to Avoid This Mistake
- Divide large classes into smaller, targeted classes.
- Distribute responsibilities among classes through composition and modular design.
- Apply the separation of concerns principle.
8. Not Using Polymorphism Effectively
Polymorphism provides flexibility by allowing multiple implementations for one interface. However, many developers overcomplicate it; moreover, they underuse its potential. Consequently, its advantages diminish, furthermore affecting maintainability.
How to Avoid This Mistake
- Develop interfaces that encourage polymorphism…
- Proper method overriding and dynamic dispatch should be followed…
- Don’t indulge in a lot of type-checking (instanceof) but rather use polymorphism instead.
9. Failing to Properly Handle Exceptions
Improper exception handling can lead to system crashes, security vulnerabilities, and difficult-to-debug applications.
How to Avoid This Mistake
- Develop interfaces that encourage polymorphism…
- Proper method overriding and dynamic dispatch should be followed…
- Don’t indulge in a lot of type-checking (instanceof) but rather use polymorphism instead.
10. Ignoring Code Readability and Maintainability
Poorly structured, hard-to-read code, consequently, causes maintenance nightmares; moreover, it increases debugging time; additionally, it delays updates; ultimately, it hampers productivity.
How to Avoid This Mistake
- Firstly, use meaningful naming conventions for classes and methods. Secondly, keep code clean and modular; and finally, ensure proper indentation and add comments.
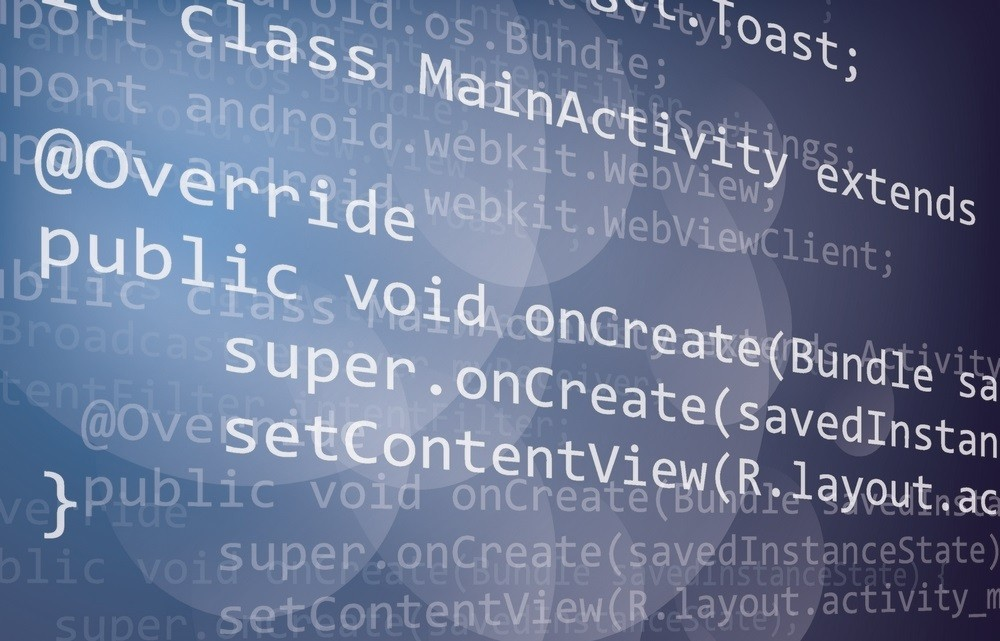
Conclusion
OOP is an organized way of doing software development, but many errors lead to inefficiency and maintenance problems. Developers actively seek robust, scalable applications; first, they adhere to SOLID principles, then embrace encapsulation, next avoid tight coupling, and finally write clean, readable code.